C#计算一段程序运行时间的三种方法
第一种方法利用System.DateTime.Now:
static
void
SubTest()
{
DateTime beforDT = System.DateTime.Now;
//耗时巨大的代码
DateTime afterDT = System.DateTime.Now;
TimeSpan ts = afterDT.Subtract(beforDT);
Console.WriteLine(
"DateTime总共花费{0}ms."
, ts.TotalMilliseconds);
}
第二种用Stopwatch类(System.Diagnostics):
static
void
SubTest()
{
Stopwatch sw =
new
Stopwatch();
sw.Start();
//耗时巨大的代码
sw.Stop();
TimeSpan ts2 = sw.Elapsed;
Console.WriteLine(
"Stopwatch总共花费{0}ms."
, ts2.TotalMilliseconds);
}
第三种用API实现:
[System.Runtime.InteropServices.DllImport(
"Kernel32.dll"
)]
static
extern
bool
QueryPerformanceCounter(
ref
long
count);
[System.Runtime.InteropServices.DllImport(
"Kernel32.dll"
)]
static
extern
bool
QueryPerformanceFrequency(
ref
long
count);
static
void
SubTest()
{
long
count = 0;
long
count1 = 0;
long
freq = 0;
double
result = 0;
QueryPerformanceFrequency(
ref
freq);
QueryPerformanceCounter(
ref
count);
//耗时巨大的代码
QueryPerformanceCounter(
ref
count1);
count = count1 - count;
result = (
double
)(count) / (
double
)freq;
Console.WriteLine(
"QueryPerformanceCounter耗时: {0} 秒"
, result);
}
也可以使用委托对其进行封装,方便调用:
/// <summary>
/// 计算时间
/// </summary>
/// <param name="function">要被执行的代码</param>
/// <returns>执行这一段代码耗时,单位:毫秒</returns>
public
static
string
Stopwatch(Action function)
{
System.Diagnostics.Stopwatch sw =
new
System.Diagnostics.Stopwatch();
sw.Start();
//开始执行业务代码
function();
sw.Stop();
TimeSpan timeSpan = sw.Elapsed;
return
(timeSpan.TotalMilliseconds) +
"ms"
;
}
版权声明:
作者:亦灵一梦
链接:https://blog.haokaikai.cn/2018/program/aspnet/139.html
来源:开心博客
文章版权归作者所有,未经允许请勿转载。
THE END
1
二维码
海报
C#计算一段程序运行时间的三种方法
第一种方法利用System.DateTime.Now:
static void SubTest()
{
DateTime beforDT = System.DateTime.Now;
//耗时巨大的代码
DateTime after……
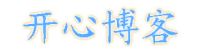