office转PDF|wps转PDF
1.0:office转图片
1.1:安装office
1.2:引用项目
Microsoft.Office.Interop.Word
Microsoft.Office.Interop.PowerPoint
microsoft.office.interop.Excel
Microsoft.Office.Core
using Word = Microsoft.Office.Interop.Word;
using Excel = Microsoft.Office.Interop.Excel;
using PowerPoint = Microsoft.Office.Interop.PowerPoint;
using Microsoft.Office.Core;
using System;
using Microsoft.Office.Interop.Excel;
using Microsoft.Office.Interop.PowerPoint;
using Microsoft.Office.Interop.Word;
1.3:
/// <summary>
/// 把Excel文件转换成PDF格式文件
/// </summary>
/// <param name="sourcePath">源文件路径</param>
/// <param name="targetPath">目标文件路径</param>
/// <returns>true=转换成功</returns>
private static bool XLSConvertToPDF(string sourcePath, string targetPath)
{
bool result = false;
Excel.XlFixedFormatType targetType = Excel.XlFixedFormatType.xlTypePDF;
object missing = Type.Missing;
Excel.Application application = null;
Excel.Workbook workBook = null;
try
{
application = new Excel.Application();
object target = targetPath;
object type = targetType;
workBook = application.Workbooks.Open(sourcePath, missing, missing, missing, missing, missing,
missing, missing, missing, missing, missing, missing, missing, missing, missing);
workBook.ExportAsFixedFormat(targetType, target, Excel.XlFixedFormatQuality.xlQualityStandard, true, false, missing, missing, missing, missing);
result = true;
}
catch
{
result = false;
}
finally
{
if (workBook != null)
{
workBook.Close(true, missing, missing);
workBook = null;
}
if (application != null)
{
application.Quit();
application = null;
}
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
GC.WaitForPendingFinalizers();
}
return result;
}
/// <summary>
/// DOC转pdf
/// </summary>
/// <param name="sourcePath"></param>
/// <param name="targetPath"></param>
/// <returns></returns>
static bool DOCConvertToPDF(string sourcePath, string targetPath)
{
bool result;
object paramMissing = Type.Missing;
Word.Application wordApplication = new Word.Application();
Document wordDocument = null;
try
{
object paramSourceDocPath = sourcePath;
string paramExportFilePath = targetPath;
Word.WdExportFormat paramExportFormat = Word.WdExportFormat.wdExportFormatPDF;
bool paramOpenAfterExport = false;
Word.WdExportOptimizeFor paramExportOptimizeFor =
Word.WdExportOptimizeFor.wdExportOptimizeForPrint;
Word.WdExportRange paramExportRange = Word.WdExportRange.wdExportAllDocument;
int paramStartPage = 0;
int paramEndPage = 0;
Word.WdExportItem paramExportItem = Word.WdExportItem.wdExportDocumentContent;
bool paramIncludeDocProps = true;
bool paramKeepIRM = true;
Word.WdExportCreateBookmarks paramCreateBookmarks =
Word.WdExportCreateBookmarks.wdExportCreateWordBookmarks;
bool paramDocStructureTags = true;
bool paramBitmapMissingFonts = true;
bool paramUseISO19005_1 = false;
wordDocument = wordApplication.Documents.Open(
ref paramSourceDocPath, ref paramMissing, ref paramMissing,
ref paramMissing, ref paramMissing, ref paramMissing,
ref paramMissing, ref paramMissing, ref paramMissing,
ref paramMissing, ref paramMissing, ref paramMissing,
ref paramMissing, ref paramMissing, ref paramMissing,
ref paramMissing);
if (wordDocument != null)
wordDocument.ExportAsFixedFormat(paramExportFilePath,
paramExportFormat, paramOpenAfterExport,
paramExportOptimizeFor, paramExportRange, paramStartPage,
paramEndPage, paramExportItem, paramIncludeDocProps,
paramKeepIRM, paramCreateBookmarks, paramDocStructureTags,
paramBitmapMissingFonts, paramUseISO19005_1,
ref paramMissing);
result = true;
}
catch (Exception e)
{
Console.WriteLine(e.Message);
result = false;
}
finally
{
if (wordDocument != null)
{
wordDocument.Close(ref paramMissing, ref paramMissing, ref paramMissing);
wordDocument = null;
}
if (wordApplication != null)
{
wordApplication.Quit(ref paramMissing, ref paramMissing, ref paramMissing);
wordApplication = null;
}
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
GC.WaitForPendingFinalizers();
}
return result;
}
/// <summary>
/// PPT转pdf
/// </summary>
/// <param name="sourcePath"></param>
/// <param name="targetPath"></param>
/// <returns></returns>
static bool PPTConvertToPDF(string sourcePath, string targetPath)
{
bool result;
PpSaveAsFileType targetFileType = PpSaveAsFileType.ppSaveAsPDF;
object missing = Type.Missing;
Microsoft.Office.Interop.PowerPoint.Application application = null;
Presentation persentation = null;
try
{
application = new Microsoft.Office.Interop.PowerPoint.Application();
persentation = application.Presentations.Open(sourcePath, MsoTriState.msoTrue, MsoTriState.msoFalse, MsoTriState.msoFalse);
persentation.SaveAs(targetPath, targetFileType, Microsoft.Office.Core.MsoTriState.msoTrue);
result = true;
}
catch (Exception e)
{
Console.WriteLine(e.Message);
result = false;
}
finally
{
if (persentation != null)
{
persentation.Close();
persentation = null;
}
if (application != null)
{
application.Quit();
application = null;
}
GC.Collect();
GC.WaitForPendingFinalizers();
GC.Collect();
GC.WaitForPendingFinalizers();
}
Console.WriteLine("ppt转pdf结束");
return result;
}
1.4:
1.5:
2.0:wps转图片
2.1:前提:需要下载安装wps专业版、企业版
2.2:项目中需要引用wps的com组件
//com组件Upgrade WPS Spreadsheets 3.0 Object Library (Beta) ,对应“Excel”,C:\WINDOWS\assembly\GAC_32\Kingsoft.Office.Interop.Etapi\3.0.0.0__15d99fb7f8fe5cb4\Kingsoft.Office.Interop.Etapi.dll
//com组件 Upgrade WPS Presentation 3.0 Object Library (Beta),对应“PowerPoint”,C:\WINDOWS\assembly\GAC_32\Kingsoft.Office.Interop.Wppapi\3.0.0.0__15d99fb7f8fe5cb4\Kingsoft.Office.Interop.Wppapi.dll
//com组件Upgrade Kingsoft WPS 3.0 Object Library (Beta),对应“Word”,C:\WINDOWS\assembly\GAC_32\Kingsoft.Office.Interop.Wpsapi\3.0.0.0__15d99fb7f8fe5cb4\Kingsoft.Office.Interop.Wpsapi.dll
2.3 转换代码
public static class WpsToPdf
{
/// <summary>
/// word转pdf
/// </summary>
/// <param name="source">源<see cref="string"/>.</param>
/// <param name="newFilePath">新文件路径<see cref="string"/>.</param>
/// <returns>The <see cref="bool"/>.</returns>
public static bool WordWpsToPdf(string source, string newFilePath)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (newFilePath == null) throw new ArgumentNullException(nameof(newFilePath));
var type = Type.GetTypeFromProgID("KWps.Application");
dynamic wps = Activator.CreateInstance(type);
try
{
//用wps打开word不显示界面
dynamic doc = wps.Documents.Open(source, Visible: false);
//转pdf
doc.ExportAsFixedFormat(newFilePath, WdExportFormat.wdExportFormatPDF);
//设置隐藏菜单栏和工具栏
//wps.setViewerPreferences(PdfWriter.HideMenubar | PdfWriter.HideToolbar);
doc.Close();
}
catch (Exception e)
{
//添加你的日志代码
return false;
}
finally
{
wps.Quit();
GC.Collect();
GC.WaitForPendingFinalizers();
}
return true;
}
/// <summary>
/// excel转pdf
/// </summary>
/// <param name="source">源<see cref="string"/>.</param>
/// <param name="newFilePath">新文件路径<see cref="string"/>.</param>
/// <returns>The <see cref="bool"/>.</returns>
public static bool ExcelToPdf(string source, string newFilePath)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (newFilePath == null) throw new ArgumentNullException(nameof(newFilePath));
var type = Type.GetTypeFromProgID("KET.Application");
dynamic wps = Activator.CreateInstance(type);
try
{
var targetType = XlFixedFormatType.xlTypePDF;
var missing = Type.Missing;
//转pdf
var doc = wps.Application.Workbooks.Open(source, missing, missing, missing, missing, missing,
missing, missing, missing, missing, missing, missing, missing, missing, missing);
doc.ExportAsFixedFormat(targetType, newFilePath, XlFixedFormatQuality.xlQualityStandard, true, false,
missing, missing, missing, missing);
doc.Close();
}
catch (Exception e)
{
//添加你的日志代码
return false;
}
finally
{
wps.Quit();
GC.Collect();
GC.WaitForPendingFinalizers();
}
return true;
}
/// <summary>
/// ppt转pdf
/// </summary>
/// <param name="source">源<see cref="string"/>.</param>
/// <param name="newFilePath">新文件路径<see cref="string"/>.</param>
/// <returns>The <see cref="bool"/>.</returns>
public static bool PptToPdf(string source, string newFilePath)
{
var type = Type.GetTypeFromProgID("KWPP.Application");
dynamic wps = Activator.CreateInstance(type);
try
{
//转pdf
var doc = wps.Presentations.Open(source, MsoTriState.msoCTrue, MsoTriState.msoCTrue,
MsoTriState.msoCTrue);
doc.SaveAs(newFilePath, PpSaveAsFileType.ppSaveAsPDF, MsoTriState.msoTrue);
doc.Close();
}
catch (Exception e)
{
//添加你的日志代码
return false;
}
finally
{
wps.Quit();
GC.Collect();
GC.WaitForPendingFinalizers();
}
return true;
}
}
版权声明:
作者:亦灵一梦
链接:https://blog.haokaikai.cn/2020/program/aspnet/984.html
来源:开心博客
文章版权归作者所有,未经允许请勿转载。
THE END
1
二维码
海报
office转PDF|wps转PDF
1.0:office转图片
1.1:安装office
1.2:引用项目
Microsoft.Office.Interop.Word
Microsoft.Office.Interop.PowerPoint
microsoft.office.interop.Excel
Mic……
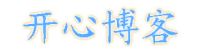